invert binary tree iterative
Leetcode June 20 challenge begins with the Tree inversion problem. In this tutorial I am going to discuss the iterative and recursive approaches to solve this problem.
1 2 5 3 4.
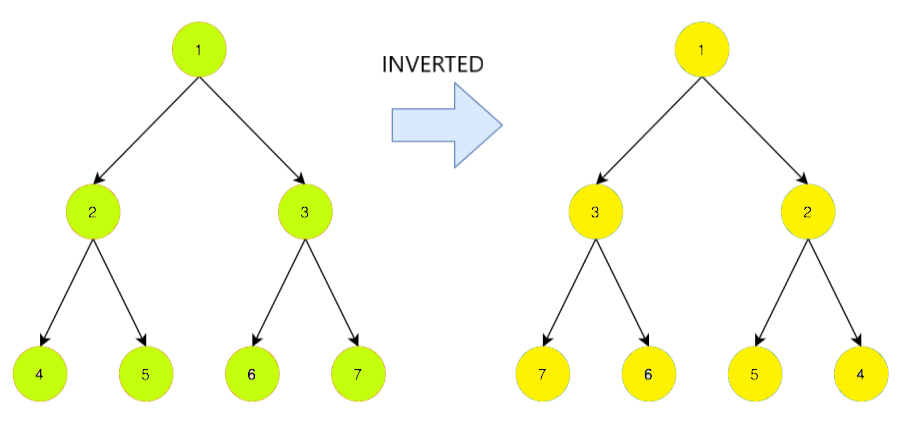
. Return None q deque node root qappend node. To solve this we will use a recursive approach. The problem is pretty simple invert tree or in other words create a mirror image of a tree.
So all we need to do is Swapping left children with right children of a binary tree at every level Implementation. Replace each node in binary tree with the sum of its inorder predecessor and successor. In simple words Output is the mirror of the input tree.
The code only changes the val fields without changing the original topology. Using Iterative preorder traversal. For converting a binary tree into its mirror tree we have to traverse a binary tree.
Below are the three approaches to solve this problem. If node is None. Inputroot 4271369Output4729631 Example 2.
Can change only the values so that in-order traversal will generate a reversed list. Create the root node. Invert a binary tree Recursive and Iterative solutions.
Invert a binary tree Recursive and Iterative solutions. Added code for inverting the Binary tree and iterative traversals. Vertical Sum in Binary Tree Set 2 Space Optimized Find root of the tree where children id sum for every node is given.
Reversing a binary tree would be what you d run in a tool to migrate all of your data over from the old format to the new format. Swapping the left and right child of every node in subtree recursively. Else if prev-data key then insert toinsert in the left of prev prev-left toinsert.
Hint 2 Once the first swap mentioned in Hint 1 is done you must invert the root nodes left child node and its right child node. 0ms C RecursiveIterative Solutions with Explanations - Leetcode Discuss. So this solution follows an approach similar to BFS where we use a queue to hold the nodes we have to iterate through.
If curr-data key set curr to be curr-left discard the right subtree. For a node say x If there exists the left child that is not yet taken then this child must become the right child of x. This looks much better with binary search trees but that.
Invert the binary tree recursively. This is the best place to expand your knowledge and get prepared for your next interview. Return None Swapping the children temp rootleft rootleft rootright rootright temp Recursion selfinvertrootleft selfinvertrootright return.
If node is None. This answer is not useful. Return root Iteratively TreeNode invertTreeTreeNode root.
Return None temp nodeleft nodeleft noderight noderight nodeleft def levelOrder root. If root None. I was working on a problem Invert Binary Tree in an iterative fashion.
You have to invert this binary tree. Hint 1 Start by inverting the root node of the Binary Tree. Store the root node in the queue and then keep on iterating the loop till the queue is not empty.
Implementation of Tree is give and is nothing different from usual containing left and right child for each node. Given a binary tree we have to invert the tree and print it. If the left child is already taken then the.
To understand the problem basic knowledge of Binary Tree is require. We discuss different approaches to solve this problem along with their time and space complexities. In simple terms it is a tree whose left children and right children of all non-leaf nodes are swapped.
Space Complexity of Invert a Binary Tree. In each iteration get the top node swap its left and right child and then add the left and right subtree back to the queue. Let n be the number of nodes in the binary tree.
Following is the code to invert a Binary Tree recursively. Treenode t n. You can do so just as you did for the root.
You can invert a binary tree using recursive and iterative approaches. Converting recursive approach to iterative by using stack. Inversion must be done by following all the below guidelines.
Show activity on this post. Show activity on this post. Then while the length of the queue is not zero we pop off the first element of the queue swap the left and right children and then append that nodes left and right child nodes.
Inverting a binary tree means we have to interchange the left and right children of all non-leaf nodes. To invert the tree iteratively Perform the level order traversal using the queue. We first create a queue with a single element the root node.
I Made This. 5 4 3 1 2. Invert Binary Tree Diameter of a Binary Tree Construct Binary Tree from Preorder and Inorder Traversal Construct Binary Tree from Inorder and Postorder Traversal.
Given a binary tree we have to write a code to invert it. Find largest subtree sum in a tree. Merge Two Binary Trees by doing Node Sum Recursive and Iterative Vertical Sum in a given Binary Tree Set 1.
Level up your coding skills and quickly land a job. Given the rootof a binary tree invert the tree and return its root. If curr-data key set curr to be curr-right discard the left subtree.
The given leaf node becomes the root after the inversion. Inverting this root node simply consists of swapping its left and right child nodes which can be done the same way as swapping two variables. The inversion of a binary tree or the invert of a binary tree means to convert the tree into its Mirror image.
Inputroot 213Output231 Example 3. TreeNode invertTreeTreeNode root ifrootNULL return NULL. In this tutorial I have explained how to invert binary tree using iterative and recursive approachLeetCode June Challenge PlayList - httpswwwyoutubeco.
If prev NULL it means that the tree is empty. So a tree that looks like.
Invert Alternate Levels Of A Perfect Binary Tree Techie Delight
Invert A Binary Tree Python Code With Example Favtutor
Invert A Binary Tree Python Code With Example Favtutor
Invert Reverse A Binary Tree 3 Methods
Invert A Binary Tree Recursive And Iterative Solutions Learnersbucket
Invert A Binary Tree Recursive And Iterative Approach In Java The Crazy Programmer
Invert A Binary Tree Python Code With Example Favtutor
Coding Short Inverting A Binary Tree In Python By Theodore Yoong Medium
Invert Binary Tree Iterative And Recursive Solution Techie Delight
Invert A Binary Tree Python Code With Example Favtutor
Convert A Binary Tree Into Its Mirror Tree Geeksforgeeks
Flip Binary Tree Geeksforgeeks
Invert Binary Tree Leetcode 226 Youtube
Algodaily Invert A Binary Tree Description
What Is The Algorithmic Approach To Invert A Given Binary Tree Quora
Invert Binary Tree Iterative Recursive Approach
Invert Reverse A Binary Tree 3 Methods
Leetcode Invert Binary Tree Solution Explained Java Youtube